티스토리 뷰
Thread 생성하기
Thread를 생성하는 방법에는 크게 두가지가 있습니다.
Thread 클래스를 상속받는 것과, Runnable 인터페이스를 구현하는 것입니다.
1. Thread 클래스
class MyThread extends Thread {
int num;
MyThread(int num) {
this.num = num;
}
@Override
public void run() {
System.out.println("# "+ num + " thread is running");
}
}
public static void main(String[] args) throws InterruptedException {
for(int i=0; i<10; i++) {
MyThread myThread = new MyThread(i);
myThread.start();
}
System.out.println("main method terminate.");
}
😀 실행 결과
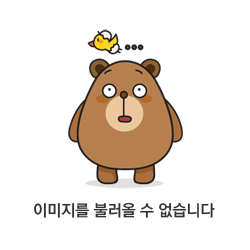
2. Runnable 인터페이스
class MyRunnable implements Runnable {
int num;
MyRunnable(int num) {
this.num = num;
}
@Override
public void run() {
System.out.println("# "+ num + " thread is running");
}
}
public static void main(String[] args) throws InterruptedException {
for(int i=0; i<10; i++) {
// Runnable은 인터페이스이기 때문에 Thread 객체로 생성합니다.
Thread myRunnable = new Thread(new MyRunnable(i));
myRunnable.start();
}
System.out.println("main method terminate.");
}
😀 실행 결과
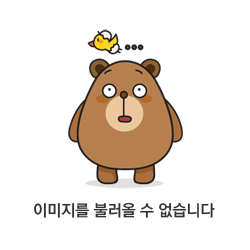
스레드 제어
위에서 만든 두 스레드를 같이 실행해보겠습니다.
public static void main(String[] args) throws InterruptedException {
for(int i=0; i<10; i++) {
MyThread myThread = new MyThread(i);
myThread.start();
Thread myRunnable = new Thread(new MyRunnable(i));
myRunnable.start();
}
System.out.println("main method terminate.");
}
😀 실행 결과
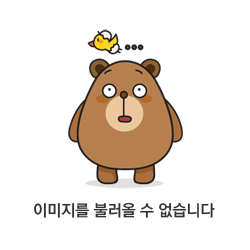
thread와 runnable이 뒤죽박죽 실행되고 있습니다.
따로 우선순위를 지정해주지 않으면 CPU의 스케줄링에 맞춰 스레드가 실행됩니다.
스레드의 실행 순서를 제어할 필요가 있을 경우 어떻게 해야할 지 알아보겠습니다.
우선순위 부여
Thread.setPriority()
메소드로 스레드에 각각의 우선순위를 부여할 수 있습니다.
public static void main(String[] args) throws InterruptedException {
MyThread myThread = new MyThread(525);
Thread myRunnable = new Thread(new MyRunnable(525));
myThread.setPriority(10);
myRunnable.setPriority(5);
myThread.start();
myRunnable.start();
System.out.println("main method terminate.");
}
😀 실행 결과
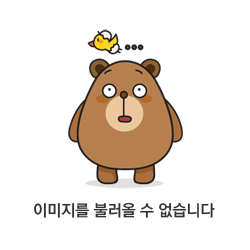
우선순위를 10으로 부여한 myThread
가 먼저 실행되고, 5로 부여한 myRunnable
은 myThread
가 종료된 후 실행됩니다.
참고로 Thread.MAX_PRIORITY
, Thread.NORM_PRIORITY
, Thread.MIN_PRIORITY
를 사용할 수도 있습니다. 각 값은 10, 5, 1 상수입니다.
join 함수
join()
메소드를 이용하면, 나중에 실행 될 스레드를 지정할 수 있습니다.
join()
을 사용하지 않았을 때
public static void main(String[] args) throws InterruptedException {
MyThread myThread = new MyThread(525);
myThread.start();
System.out.println("main method terminate.");
}
}
😀 실행 결과
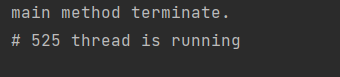
스레드의 실행여부와 관계 없이 메인 메소드가 끝나버립니다.
join()
을 사용했을 때
public static void main(String[] args) throws InterruptedException {
MyThread myThread = new MyThread(525);
myThread.start();
myThread.join();
System.out.println("main method terminate.");
}
😀 실행 결과
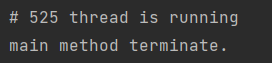
메인 메소드가 스레드가 끝날 때까지 기다려줍니다.join()
메소드를 호출하면, 호출한 스레드 (main
)은 waiting 상태로 들어가게 되고,
호출당한 스레드(myThread
)가 종료할 때까지 기다린다는 것을 알 수 있습니다.
- Total
- Today
- Yesterday
- 자바
- greedy
- 시퀀스가존재하지않습니다
- 백준1976
- effectivejava
- 트리순회
- 빌더패턴
- Sequence
- 전위순회
- 이펙티브자바
- 스레드
- 분리집합
- 여행가자
- Java
- 후위순회
- ORA-02289
- 유니온파인드
- deque
- 투포인터 #알고리즘
- 이진트리
- 시퀀스
- BuilderPattern
- 프로그래머스
- 중위순회
- BAEKJOON
- 생성자
- 탐욕법
- 알고리즘
- 정적팩터리메서드
- 백준
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |